# Endpoints
This page describes use cases and endpoints around the anybill Partner Platform API. The Partner Platform API is used to allow integrations with third party partners in multiple use cases.
- Receive receipts in frontend application (Mobile, Web)
- Receive receipts by loyalty/customer cards
- Link user base with anybill accounts
- Automatic onboarding of customers/merchants within the POS system
- Check onboarding status of customers/merchants from the POS system
- Retrieve list of user receipts with minimal receipt information in combination with the Receipt Notification Webhook
- Generate PDF for user receipt
- Add anonymous receipt to user
# User Endpoints
To enable your users to access their anybill receipts without the need for them to create dedicated anybill accounts, our anybill Partner Platform API offers a convenient solution.
We offer an endpoint that allows your organization to create anonymous anybill users. These anonymous users are exempt from the typical registration process, eliminating the need to fill out login information and other associated details. Instead, your organization can effortlessly create anonymous anybill user profiles on behalf of your users, granting them seamless access to anybill services.
These anonymous anybill users enable the interaction with our anybill Frontend SDKs, which encompasses the core functionalities of our platform, including the ability to add, receive and manage bills and perform various other essential tasks.
# Basic Workflow
The workflow for creating an anonymous anybill user works as following:
- Create an anonymous user using the create endpoint (opens new window)
- Save the returned
userId
to your current user model as e.g.anybillUserId
- Request token information using the
anybillUserId
and the token endpoint (opens new window) - Forward the token information to your frontend application and the anybill Frontend application to authenticate the user and enable access to the anybill functions in the frontend
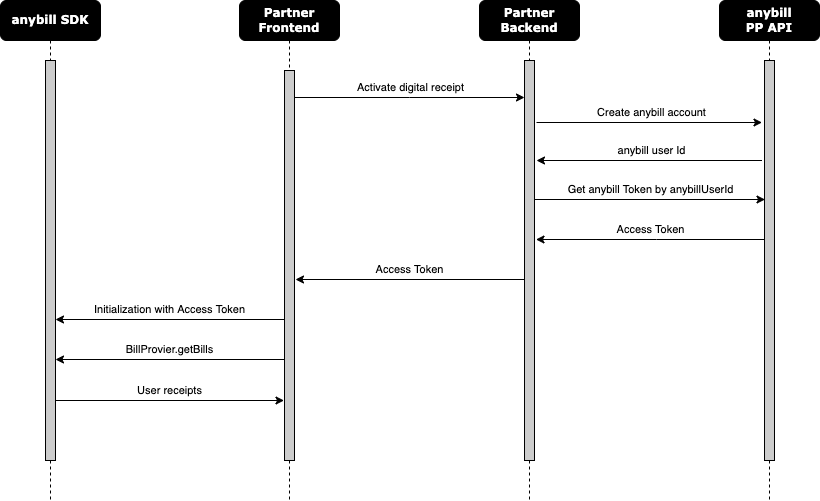
# Create an anonymous account
The create user endpoint can be used to create anonymous anybill user and link them to an existing customer account within your organisation.
To create an anonymous anybill account call the POST /api/v3/user
endpoint (opens new window).
To enable the automatic receival of receipts by scanning a customer or loyalty cards, an identifier has to be provided during user creation as externalId
. This identifier can be any type of ID (e.g. customer number, card number, userId, e-mail)
Identifier
- The identifier has to be unique within your organization
- The identifier has to be present at the Point of Sale either by being scanned directly or by a Backend Integration between your organisation and the POS (for receipt receival with loyalty cards)
Request
{
"externalId": "<identifier>"
}
Response
{
"id": "9632d033-b25d-47c0-8aa9-85babec64969"
}
The returned id
represents the userId of the newly created anybill user. Store this ID in your existing user model and use it to request authentication token for your frontend application using the token endpoint. (opens new window)
# Multiple loyalty cards
Additionally if multiple loyalty cards are in use, a user can be created with additional identifiers using the loyaltycards
property.The loyalty cards will be associated to the vendor creating the user and containing the provided barcodes. The property loyaltyCards
can contain multiple loyalty cards for one user. Loyalty cards can be managed with the /api/v3/user/{userId}/loyaltycard
endpoints (opens new window).
{
"externalId": "customerNumber",
"loyaltyCards": [
{
"code": "123456789",
"name": "PartnerCustomerCard"
},
{
"code": "1234567890",
"name": "AdditionalLoyaltyCard"
}
]
}
# Retrieving token information for an anybill account
To authenticate an anybill user within the anybill SDK in your frontend application, you'll have to retrieve token information using the obtained user Id during user creation. The token should then be forwarded to your frontend application and used to initialize the anybill SDK.
Request:
userId: anybill userId obtained during user creation
applicationClientId (optional): Client-Id of your frontend application which is requesting the token
GET api/v3/user/{userId}/token?applicationClientId={applicationClientId}
Multiple frontend application
If you are handling multiple frontend applications with one Partner Platform API integration, setting the applicationClientId is essential to differ between the requesting frontend applications. This is useful for error handling, data analysis and to return different data between your applications (e.g. stores).
Response Body:
{
"id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"accessToken": "string",
"refreshToken": "string",
"expiresIn": "string"
}
accessToken: Token information which should be forwarded to the frontend application
refreshToken: Token information which should be forwarded to the frontend application
expiresIn: Token information which should be forwarded to the frontend application
404 Error - user-temporarily-not-available
During user creation an anonymous user is created in our Azure B2C. Occasionally the user creation can take up to 1 minute. If you are create an anybill user and request a token immediately, you might receive an error as response (Currently we are observing this error at 1/500 user creations).
For this case, the Token endpoint returns a 404
Error with type user-temporarily-not-available
. You can check for this error by parsing the response error and comparing ProblemDetails.Type
:
{
"type": "user-temporarily-not-available",
"title": "Not Found",
"status": 404,
"detail": "string",
"instance": "string",
"additionalProp1": "string",
"additionalProp2": "string",
"additionalProp3": "string"
}
To handle the error you can either implement multiple retries or display an error message in your frontend, which indicates the user to wait for 1-2 minutes.
# Deleting an anonymous account
If your anybill account is not needed anymore you can delete it using the DELETE /api/user/{userId}
endpoint (opens new window).
# Onboarding Endpoints
anybill provides the possibility to automatically onboard a merchant, by generating Vendor API credentials and a merchant account based on the provided merchant information. Automatic onboarding can either be used from a server client containing merchant information or directly from the POS.
The onboarding controller contains two endpoints which can be used to create a new customer as a POS provider and check the current status of the customer.
# Onboarding a merchant
- POST
onboarding
Send master data to the anybill system for automatic onboarding
{
"vendorCustomer": {
// Information about the merchant
},
"stores": [
// Information about the stores of the merchant (Optional)
],
"invoicingDetails": {
// Information about the prefered invoicing of the merchant
}
}
# Onboarding from a server client
If your merchant data (address, name, stores, billing) is stored in an available cloud system, the merchant can be enrolled by your backend using the server client variant. This way the merchant is enrolled by providing all necessary data. Your system is going to receive a registration link for the merchant and Vendor API credentials which have to be forwarded to the POS (automatically or manual).
# Onboarding directly from the POS
If your merchant data isn't available beforehand (e.g. when merchants set up their POS system themselves) the Onboarding Endpoint can also be called direclty from the POS during initial setup (or initial activation of digital receipts). This way the registration link can be directly displayed on the POS and the received credentials can be stored withing the POS.
Enrolling stores automatically
This endpoint can be used either from a server client containing all the merchant and store information or directly from a POS. Calling it from a POS without information about other stores requires integration of the store endpoint of the Vendor API (opens new window).
# Check customer's onboarding status
GET
onboarding/vendorCustomerId/{vendorCustomerId}
Customer is activatedIf the customer finished the onboarding successfull, the HTTP status code
200 Ok
is returned with aCustomerActivatedDto
response.
{
"type": "activated",
"customerId": "3fa85f64-5717-4562-b3fc-2c963f66afa6"
}
- If the customer did not finish the onboarding, the HTTP status code
200 Ok
is returned with aCustomerNotActivatedDto
response. This means that the customer can not send receipts via the bill endpoints.
{
"type": "notActivated",
"customerId": "3fa85f64-5717-4562-b3fc-2c963f66afa6"
}
# Detailed Endpoint Description
- For Staging Environment: SwaggerUI (opens new window)
- For Production Environment: SwaggerUI (opens new window)
# Receipt Endpoints
Additionally to the creation of anybill users and the enabling of receipt by loyality card the Partner Platform API provides endpoints to query user receipts and export them as PDF. We recommend these endpoints for customers in combination with the receipt notitication webhook and costumers without the possibility to integrate the anybill SDK.
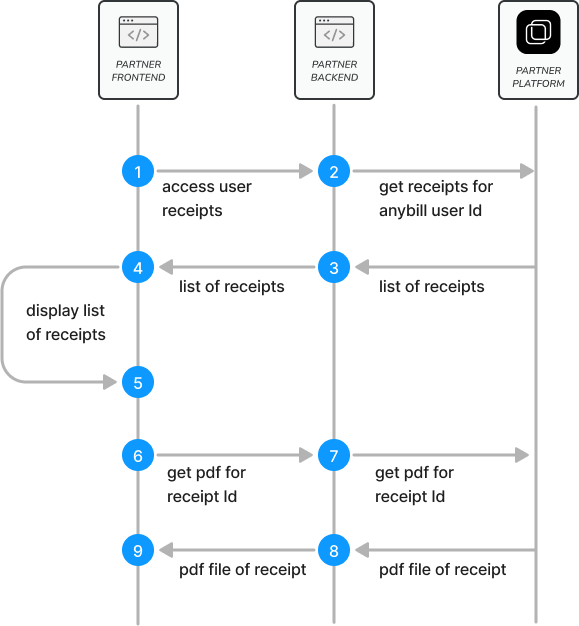
Receipt Data
The Partner Platform API endpoints provide a reduces receipts model with relevant data to be displayed in a receipt list in a frontend application. For detailed receipt information or additional features, the anybill SDK has to be integrated in frontend applications.
# Retrieve list of receipts for user
The receipt retrieval endpoint returns a list of reciept for a specific anybill user, to be displayed in a paginated list in the frontend application. To register an anybill account use the user endpoints.
# Request Parameters
Parameter Name | Value |
---|---|
userId | <id> |
take | 100 |
continuation | <token> |
# Response Headers
Header Name | Value |
---|---|
Total-Count | 35 |
ContinuationToken | <token> |
If the amount of receipts of the user exceed the page size ("take" parameter) a continuation token is returned in the Headers of the response. Use this continuation token in the following request to query the next page of receipts.
# Response Example
[
{
"receiptId": "bc5085a0-4b14-4cac-8ae3-c5561e7c76ae",
"storeId": "ea29b33a-21a6-4276-b330-415c806b87a2",
"amount": 150.0,
"currency": "EUR",
"date": "2024-02-27T16:18:14.5849630+01:00",
"imageResource": "https://anybillqa.blob.core.windows.net/stores/49fe5cae-cf28-4cbb-84bc-a1c5551d9f12.png",
"vendorName": "Lieblingsladen",
"vendorAddress": {
"street": "Sandstraße 33",
"city": "München",
"postalCode": "80335",
"countryCode": "DEU"
}
},
{
"receiptId": "2de34445-c2fe-4829-8768-48e91224ea47",
"storeId": "ea29b33a-21a6-4276-b330-415c806b87a2",
"amount": 22.52,
"currency": "EUR",
"date": "2024-02-29T15:55:20.5567630+01:00",
"imageResource": null,
"vendorName": "Bäckerei",
"vendorAddress": {
"street": "Franz Mayer Straße 1",
"city": "Regensburg",
"postalCode": "93053",
"countryCode": "Deutschland"
}
}
]
# Generate PDF File for receipt
To access the original receipt as PDF file call the PDF generation endpoint using a receiptId and the corresponding userId:
# Request Parameters
Parameter Name | Value |
---|---|
userId | <id> |
receiptId | <id> |
# Add anonymous receipt to user
To enable the acquisition of frontend users through digital receipts anybill provides the possibility to link out into frontend apps from the receipt retrieval website. During the link out the receiptId of the receipt is forwarded to your frontend application and can be used to add the receipt to an registered anybill user.
user registration
Before being able to add an anonymous receipt, the anybill user has to be registered using the user endpoints.
# Example Request
Parameter Name | Value |
---|---|
userId | <id> |
{
"receiptId": "bc5085a0-4b14-4cac-8ae3-c5561e7c76ae",
}
# Example Response
"bc5085a0-4b14-4cac-8ae3-c5561e7c76ae"
# Detailed Endpoint Description
- For Staging Environment: SwaggerUI (opens new window)
- For Production Environment: SwaggerUI (opens new window)